Blinking: Difference between revisions
m TheKillerBunny moved page User:TheKillerBunny/Blink to Blinking |
mNo edit summary |
||
Line 1: | Line 1: | ||
First, you need to have eyes to scale. I'm gonna store them in a variable for easier accessing, as well as how fast I want to blink (in ticks) and the height of my eyes in blockbench units. Your path will be different | First, you need to have eyes to scale. I'm gonna store them in a variable for easier accessing, as well as how fast I want to blink (in ticks) and the height of my eyes in blockbench units. Your path will be different | ||
[[File:EyeGroup.png|thumb]] | [[File:EyeGroup.png|thumb]] |
Revision as of 14:02, 27 September 2024
First, you need to have eyes to scale. I'm gonna store them in a variable for easier accessing, as well as how fast I want to blink (in ticks) and the height of my eyes in blockbench units. Your path will be different
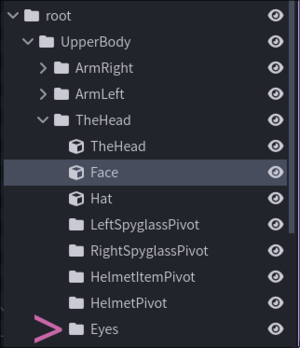
local eyes = models.model.root.UpperBody.TheHead.Eyes
local EYE_HEIGHT = 2 --The height of your eyes in blockbench units
local BLINK_RATE = 4 * 20 -- 4 Seconds
Next, I want to create a TICK
event to set when my eyes blink, as well as 5 variables: tick, oldScale, newScale, oldPos, and newPos, initialized as shown
local oldScale = vec(1, 1, 1)
local newScale = vec(1, 1, 1)
local oldPos = vec(0, 0, 0)
local newPos = vec(0, 0, 0)
local tick = 0
function events.TICK()
-- Sets old pos and scale for later interpolation
oldPos = newPos
oldScale = newScale
tick = tick + 1
-- If time to blink, set new position and scale to close the eyes, otherwise open them
if tick % BLINK_RATE == 0 then
newScale = vec(1, 0, 1)
newPos = vec(0, EYE_HEIGHT / -2, 0)
else
newScale = vec(1, 1, 1)
newPos = vec(0, 0, 0)
end
end
Finally, you want to create a RENDER
event to make the blinking smooth
function events.RENDER(delta)
-- This interpolates the blinking to make for a smooth blink
local scale = math.lerp(oldScale, newScale, delta)
local pos = math.lerp(oldPos, newPos, delta)
eyes:setPos(pos):setScale(scale)
end